728x90
반응형
기본 코드 :
//더하기 메소드
static public Matrix Plus(Matrix m1, Matrix m2) {
Matrix result;
/*
행렬의 크기들을 비교하여 더 작은 행과 열에 맞추어 계산한다.
Ex) 1 2 3 3 4 4 6 3
4 5 6 + 1 2 = 5 7 6
7 8 9 7 8 9
*/
if(m1.row > m2.row && m1.column > m2.column) {
result = PlusMatrix(m1, m2, m2.row, m2.column);
}
else if(m1.row <= m2.row) {
result = PlusMatrix(m1, m2, m1.row, m2.column);
}
else if(m1.column <= m2.column) {
result = PlusMatrix(m1, m2, m2.row, m1.column);
}
else {
result = PlusMatrix(m1, m2, m1.row, m1.column);
}
return result;
}
//빼기 메소드
/*
행렬의 크기들을 비교하여 더 작은 행과 열에 맞추어 계산한다.
Ex) 1 2 3 3 4 -2 -2 3
4 5 6 - 1 2 = 3 3 6
7 8 9 7 8 9
*/
static public Matrix Minus(Matrix m1, Matrix m2) {
Matrix result;
if(m1.row > m2.row && m1.column > m2.column) {
result = MinusMatrix(m1, m2, m2.row, m2.column);
}
else if(m1.row <= m2.row) {
result = MinusMatrix(m1, m2, m1.row, m2.column);
}
else if(m1.column <= m2.column) {
result = MinusMatrix(m1, m2, m2.row, m1.column);
}
else {
result = MinusMatrix(m1, m2, m1.row, m1.column);
}
return result;
}
static private Matrix PlusMatrix(Matrix m1, Matrix m2, int row, int column) {
/*
반복되는 코드들을 간추려 메소드화 시켰다.
*/
Matrix res = new Matrix(row, column);
for(int i = 0; i < row; i++) {
ArrayList m1_row = (ArrayList) m1.matrix[i];
ArrayList m2_row = (ArrayList) m2.matrix[i];
ArrayList columns = new ArrayList();
for(int j = 0; j < column; j++) {
columns.Add((int)m1_row[j] + (int)m2_row[j]);
}
res.matrix.Add(columns);
}
return res;
}
static private Matrix MinusMatrix(Matrix m1, Matrix m2, int row, int column) {
/*
반복되는 코드들을 간추려 메소드화 시켰다.
*/
Matrix res = new Matrix(row, column);
for(int i = 0; i < row; i++) {
ArrayList m1_row = (ArrayList) m1.matrix[i];
ArrayList m2_row = (ArrayList) m2.matrix[i];
ArrayList columns = new ArrayList();
for(int j = 0; j < column; j++) {
columns.Add((int)m1_row[j] - (int)m2_row[j]);
}
res.matrix.Add(columns);
}
return res;
}
Plus와 Minus코드가 column.Add() 부분의 아주 약간의 차이를 빼곤 차이가 없기에 쓸데없는 코드 반복을 줄이기 위해
대리자 연산자를 사용해서 줄여보고자 한다.
변경된 코드 :
delegate int PlusMinusCalc(int a, int b);
static public Matrix CalcMatrix (Matrix m1, Matrix m2,
PlusMinusCalc pmc) {
Matrix result;
if(m1.row > m2.row && m1.column > m2.column) {
result = CalculatePlusMinus(m1, m2, m2.row, m2.column, pmc);
}
else if(m1.row <= m2.row) {
result = CalculatePlusMinus(m1, m2, m1.row, m2.column, pmc);
}
else if(m1.column <= m2.column) {
result = CalculatePlusMinus(m1, m2, m2.row, m1.column, pmc);
}
else {
result = CalculatePlusMinus(m1, m2, m1.row, m1.column, pmc);
}
return result;
}
static private Matrix CalculatePlusMinus (Matrix m1, Matrix m2, int row,
int column, PlusMinusCalc pmc) {
/*
반복되는 코드들을 간추려 메소드화 시켰다.
*/
Matrix res = new Matrix(row, column);
for(int i = 0; i < row; i++) {
ArrayList m1_row = (ArrayList) m1.matrix[i];
ArrayList m2_row = (ArrayList) m2.matrix[i];
ArrayList columns = new ArrayList();
for(int j = 0; j < column; j++) {
columns.Add(pmc((int)m1_row[j], (int)m2_row[j]));
}
res.matrix.Add(columns);
}
return res;
}
class main {
static public int PlusDelegate(int a, int b) {return a + b;}
static public int MinusDelegate(int a, int b) {return a - b;}
static void Main() {
Matrix m1 = new Matrix();
m1.inputRowColumn();
m1.inputMatrix();
m1.printMatrix();
Matrix m2 = new Matrix();
m2.inputRowColumn();
m2.inputMatrix();
m2.printMatrix();
Matrix m3 = Matrix.CalcMatrix(m1, m2, new PlusMinusCalc(PlusDelegate));
m3.printMatrix();
Matrix m4 = Matrix.CalcMatrix(m1, m2, new PlusMinusCalc(MinusDelegate));
m4.printMatrix();
}
}
1. 대리자 연산자를 정의한다.
delegate int PlusMinusCalc(int a, int b);
2. 연산에 필요한 메소드들을 정의한다.
static public int PlusDelegate(int a, int b) {return a + b;}
static public int MinusDelegate(int a, int b) {return a - b;}
3. 필요한 메소드에 대리자 연산자를 매개변수로 넣는다.
static public Matrix CalcMatrix (Matrix m1, Matrix m2,
PlusMinusCalc pmc) {
···
}
static private Matrix CalculatePlusMinus
(Matrix m1, Matrix m2, int row,
int column, PlusMinusCalc pmc) {
/*
반복되는 코드들을 간추려 메소드화 시켰다.
*/
···
}
4. 메소드 호출시 대리자 연산자를 생성하여 매개변수로 보낸다.
//Plus 연산
Matrix m3 = Matrix.CalcMatrix(m1, m2, new PlusMinusCalc(PlusDelegate));
m3.printMatrix();
//Minus 연산
Matrix m4 = Matrix.CalcMatrix(m1, m2, new PlusMinusCalc(MinusDelegate));
m4.printMatrix();
사용 예제 :
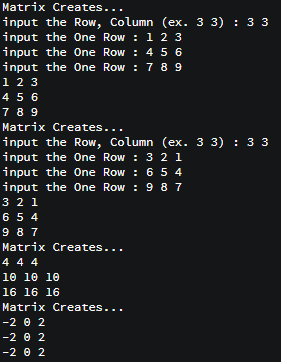
728x90
반응형
'제작 > 기타 프로그램' 카테고리의 다른 글
[C#] 행렬 계산기 (6) - 전체 코드 (0) | 2020.10.21 |
---|---|
[C#] 행렬 계산기 (5) - 곱셈 계산 (0) | 2020.10.21 |
[C#] 행렬 계산기 (4) - 이차원 배열로 더 간단하게 표현 (0) | 2020.10.20 |
[C#] 행렬 계산기(2) - 더하기, 빼기 메소드 (0) | 2020.10.11 |
[C#] 행렬 계산기 (1) - 행렬 생성 및 입력 출력 (0) | 2020.10.09 |
728x90
반응형
기본 코드 :
//더하기 메소드
static public Matrix Plus(Matrix m1, Matrix m2) {
Matrix result;
/*
행렬의 크기들을 비교하여 더 작은 행과 열에 맞추어 계산한다.
Ex) 1 2 3 3 4 4 6 3
4 5 6 + 1 2 = 5 7 6
7 8 9 7 8 9
*/
if(m1.row > m2.row && m1.column > m2.column) {
result = PlusMatrix(m1, m2, m2.row, m2.column);
}
else if(m1.row <= m2.row) {
result = PlusMatrix(m1, m2, m1.row, m2.column);
}
else if(m1.column <= m2.column) {
result = PlusMatrix(m1, m2, m2.row, m1.column);
}
else {
result = PlusMatrix(m1, m2, m1.row, m1.column);
}
return result;
}
//빼기 메소드
/*
행렬의 크기들을 비교하여 더 작은 행과 열에 맞추어 계산한다.
Ex) 1 2 3 3 4 -2 -2 3
4 5 6 - 1 2 = 3 3 6
7 8 9 7 8 9
*/
static public Matrix Minus(Matrix m1, Matrix m2) {
Matrix result;
if(m1.row > m2.row && m1.column > m2.column) {
result = MinusMatrix(m1, m2, m2.row, m2.column);
}
else if(m1.row <= m2.row) {
result = MinusMatrix(m1, m2, m1.row, m2.column);
}
else if(m1.column <= m2.column) {
result = MinusMatrix(m1, m2, m2.row, m1.column);
}
else {
result = MinusMatrix(m1, m2, m1.row, m1.column);
}
return result;
}
static private Matrix PlusMatrix(Matrix m1, Matrix m2, int row, int column) {
/*
반복되는 코드들을 간추려 메소드화 시켰다.
*/
Matrix res = new Matrix(row, column);
for(int i = 0; i < row; i++) {
ArrayList m1_row = (ArrayList) m1.matrix[i];
ArrayList m2_row = (ArrayList) m2.matrix[i];
ArrayList columns = new ArrayList();
for(int j = 0; j < column; j++) {
columns.Add((int)m1_row[j] + (int)m2_row[j]);
}
res.matrix.Add(columns);
}
return res;
}
static private Matrix MinusMatrix(Matrix m1, Matrix m2, int row, int column) {
/*
반복되는 코드들을 간추려 메소드화 시켰다.
*/
Matrix res = new Matrix(row, column);
for(int i = 0; i < row; i++) {
ArrayList m1_row = (ArrayList) m1.matrix[i];
ArrayList m2_row = (ArrayList) m2.matrix[i];
ArrayList columns = new ArrayList();
for(int j = 0; j < column; j++) {
columns.Add((int)m1_row[j] - (int)m2_row[j]);
}
res.matrix.Add(columns);
}
return res;
}
Plus와 Minus코드가 column.Add() 부분의 아주 약간의 차이를 빼곤 차이가 없기에 쓸데없는 코드 반복을 줄이기 위해
대리자 연산자를 사용해서 줄여보고자 한다.
변경된 코드 :
delegate int PlusMinusCalc(int a, int b);
static public Matrix CalcMatrix (Matrix m1, Matrix m2,
PlusMinusCalc pmc) {
Matrix result;
if(m1.row > m2.row && m1.column > m2.column) {
result = CalculatePlusMinus(m1, m2, m2.row, m2.column, pmc);
}
else if(m1.row <= m2.row) {
result = CalculatePlusMinus(m1, m2, m1.row, m2.column, pmc);
}
else if(m1.column <= m2.column) {
result = CalculatePlusMinus(m1, m2, m2.row, m1.column, pmc);
}
else {
result = CalculatePlusMinus(m1, m2, m1.row, m1.column, pmc);
}
return result;
}
static private Matrix CalculatePlusMinus (Matrix m1, Matrix m2, int row,
int column, PlusMinusCalc pmc) {
/*
반복되는 코드들을 간추려 메소드화 시켰다.
*/
Matrix res = new Matrix(row, column);
for(int i = 0; i < row; i++) {
ArrayList m1_row = (ArrayList) m1.matrix[i];
ArrayList m2_row = (ArrayList) m2.matrix[i];
ArrayList columns = new ArrayList();
for(int j = 0; j < column; j++) {
columns.Add(pmc((int)m1_row[j], (int)m2_row[j]));
}
res.matrix.Add(columns);
}
return res;
}
class main {
static public int PlusDelegate(int a, int b) {return a + b;}
static public int MinusDelegate(int a, int b) {return a - b;}
static void Main() {
Matrix m1 = new Matrix();
m1.inputRowColumn();
m1.inputMatrix();
m1.printMatrix();
Matrix m2 = new Matrix();
m2.inputRowColumn();
m2.inputMatrix();
m2.printMatrix();
Matrix m3 = Matrix.CalcMatrix(m1, m2, new PlusMinusCalc(PlusDelegate));
m3.printMatrix();
Matrix m4 = Matrix.CalcMatrix(m1, m2, new PlusMinusCalc(MinusDelegate));
m4.printMatrix();
}
}
1. 대리자 연산자를 정의한다.
delegate int PlusMinusCalc(int a, int b);
2. 연산에 필요한 메소드들을 정의한다.
static public int PlusDelegate(int a, int b) {return a + b;}
static public int MinusDelegate(int a, int b) {return a - b;}
3. 필요한 메소드에 대리자 연산자를 매개변수로 넣는다.
static public Matrix CalcMatrix (Matrix m1, Matrix m2,
PlusMinusCalc pmc) {
···
}
static private Matrix CalculatePlusMinus
(Matrix m1, Matrix m2, int row,
int column, PlusMinusCalc pmc) {
/*
반복되는 코드들을 간추려 메소드화 시켰다.
*/
···
}
4. 메소드 호출시 대리자 연산자를 생성하여 매개변수로 보낸다.
//Plus 연산
Matrix m3 = Matrix.CalcMatrix(m1, m2, new PlusMinusCalc(PlusDelegate));
m3.printMatrix();
//Minus 연산
Matrix m4 = Matrix.CalcMatrix(m1, m2, new PlusMinusCalc(MinusDelegate));
m4.printMatrix();
사용 예제 :
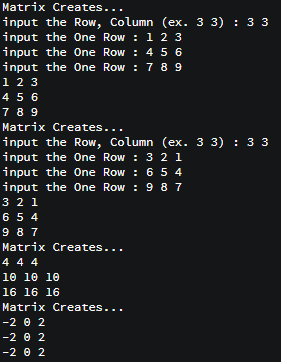
728x90
반응형
'제작 > 기타 프로그램' 카테고리의 다른 글
[C#] 행렬 계산기 (6) - 전체 코드 (0) | 2020.10.21 |
---|---|
[C#] 행렬 계산기 (5) - 곱셈 계산 (0) | 2020.10.21 |
[C#] 행렬 계산기 (4) - 이차원 배열로 더 간단하게 표현 (0) | 2020.10.20 |
[C#] 행렬 계산기(2) - 더하기, 빼기 메소드 (0) | 2020.10.11 |
[C#] 행렬 계산기 (1) - 행렬 생성 및 입력 출력 (0) | 2020.10.09 |